F-string in Python: An Easy and Comprehensive Tutorial
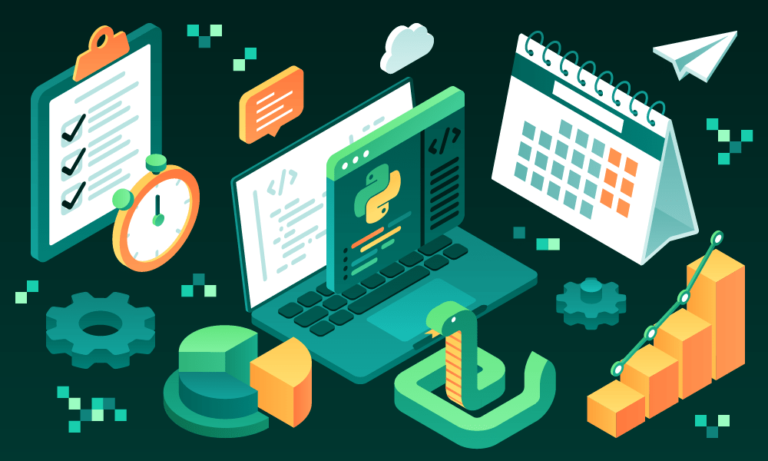
Life, they say, is a steady progression. Once upon a time, developers and programmers alike had to put up with two primitive ways of python formatting: % formatting and str.format(). Those were dark times and writing codes were possibly the most daunting task at that time. Then suddenly, it was 2015, and Python 3.6 was introduced and, with it, python f string. The new python fstring didn’t only make writing codes easy and fast, it also made it possible for developers to write codes that can carry embedded expressions, simpler syntaxes as well as work with incredible speed at runtime.
What is F-string Formatting
Before we find out what f-string formatting is, we are sure you are asking, what is an f string? F string is short for “formatted string.” It is a literal in python programming language that comes with the letter “f” at the beginning as well as curly braces that contain expressions. We can later replace these expressions with their values within the curly braces.
F-string formatting, therefore, means formatting a string of code by simply prefixing it with the letter “f”, and then including curly braces that will contain the expressions. Including the expressions within the curly braces allows the developer greater control over the code.
F-string formatting is a favorite for many developers because of so many reasons. Some of them include:
- It Has a simpler syntax
The syntax used in f string formatting looks almost like the type used in str.format() but it is more readable. The string is also minimal with fewer lines. However, ‘\’, and ‘#’ are not allowed to be part of the expressions in fstring python. There is more information on this and more in the python f-string documentation.
- It is Fast, Flexible, and Concise
Since f-strings work with runtime evaluations rather than a constant value, there is every tendency that the code would run swiftly and output would be returned in record time. Working with f strings isn’t only flexible and concise but it is also faster than what can be attained with other programming languages.
- It Has Arbitrary Expressions/Variables that are Evaluated in Runtime
F-string supports numerous variables and virtually all expressions found on python can be used on it. The variables include strings, lists, integers, dictionaries, and tuples. Also, the expressions are usually evaluated in runtime which ensures fewer errors when compared with older ways of formatting.
- It Allows for Multi-line strings
Another reason why python f-string is loved is because of how it offers the chance to include multiple lines of strings within a piece of code. This can be done very easily in two ways; by using triple quotes or by using the newline (\n) command to signal the creation of a new line. However, to ensure the output comes out as intended, we must remember to use the letter “f” as the prefix of each line as failing to do so would result in something else.
Distinct Features of F-string Formatting in Python
Aside from the numerous advantages that f string formatting in python has over other types of formatting, many features are unique to f-string formatting alone. Some of these features are explained below;
- The Use of Quotation Marks
The first distinct feature you would notice about f string python is how we are allowed to use quotations within expressions. Be it single (‘.’), double (“.”), or triple (‘’’.’’’) quotes. There are only two rules here; end with the same quotation mark you start with and do not use the same type of quotation mark inside and outside the f-string.
Let us see examples of f string python codes with different quotation marks.
With single quotes:
>>> f"{'Kelvin Klein'}"
'Kelvin Klein'
With double quotes:
>>> f'{"Kelvin Klein"}'
'Kelvin Klein'
With triple quotes:
>>> f"""Kelvin Klein"""
'Kelvin Klein'
>>> f'''Kelvin Klein'''
'Kelvin Klein'
2. The Use of Dictionaries
The use of dictionaries is also another unique feature of f-string formatting. The dictionary usually contains the values and any other python string variable we may be working with. But for this feature to work correctly, we have to remember to use single quotes around the dictionary variables and double quotes around the f-string. Failure to do so will result in a syntax error.
See below a piece of code containing a dictionary correctly written:
>>> designer = {'name': 'Kelvin Klein', 'age': 86}
>>> f"The designer is {designer['name']}, aged {designer['age']}."
The designer is Kelvin Klein, aged 86.
And below is a piece of code containing a dictionary but with the wrong quotes. We see that it results in a SyntaxError:
>>> designer = {'name': 'Kelvin Klein', 'age': 86}
>>> f'The designer is {designer['name']}, aged {designer['age']}.'
File "<stdin>", line 1
f'The designer is {designer['name']}, aged {designer['age']}.'
SyntaxError: invalid syntax
3. The Use of Curly Braces
It is also possible to produce a python output with braces. This is something that can only be seen with f-string. To see curly braces in the output, simply use double braces in the code as shown below:
>>> f"{{8 + 4}}"
'{8 + 4}'
If you want to see your output enclosed inside double curly braces, then you need to use four curly braces on each side in your code. Like what you see below:
>>> f"{{{{8 + 4}}}}"
'{{12}}'
4. The Use of Backlashes
This another unique feature of python fstring formatting. The backslash (\) is usually used to escape one line to a new line. It is worthy to note that the backlashes cannot be used inside the curly braces that contain our expression. It can only be used outside the braces, that is, in the f-string part of the code. To do otherwise would result in SyntaxError as seen below:
>>> f"{\"Kelvin Klein\"}"
File "<stdin>", line 1
f"{\"Kelvin Klein\"}"
^
SyntaxError: f-string expression part cannot include a backslash
5. The Use of Inline Comments
Inline comments are also a thing with python f-strings. They allow us to make little comments about our lines of code. You can even call them “code explanations” if you like. To include an inline comment, simply put a hashtag sign (#) above the line of code you wish to comment on and then type your comment next to it. But you should never include either the inline comment within the expression as that would result in an error as seen in the code below:
>> f"Kelvin Klein is {2 * 43 #To multiply his age!}."
File "<stdin>", line 1
f"Kelvin Klein is {2 * 43 # To multiply his age!}."
^
SyntaxError: f-string expression part cannot include '#'
Examples of Working with F-string in Python
The following are examples of working with f-string formatting in python.
Example 1. A simple code returning a simple python formatting output:
>>> name = 'Kelvin Klein'
>>> age = 86
>>> f'He said his name is {name} and he is {age} years old.'
He said his name is Kelvin Klein and he is 86 years old.
Example 2: A code showing multiline strings:
>>> name = "Kelvin Klein"
>>> profession = "designer"
>>> affiliation = "Fashion Magazine"
>>> message = (
... f"Hi {name}. "
... f"You are a {profession}. "
... f"You were in {affiliation}."
... )
>>> message
'Hi, Kelvin Klein. You are a designer. You were in Fashion Magazine.'
Example 3: A python evaluates string showing the result of today’s date:
# Prints today’s date with the help
# of datetime library
import datetime
today = datetime.datetime.today()
print(f”{today:%B %d, %Y}”)
For this, the python formatting output would be:
November 14, 2020
Pitfalls of Python F String and Their Solutions
Compared to other methods of formatting, python f strings are inarguably the most preferred choice. This is especially because of its simple syntax as well as the speed with which it can be evaluated. However, this is not to say there are no downsides to using f string formatting. Let us look at a few of its pitfalls as well their solutions
- Only Compactible with Python 3.6 and Above
Python f string format in all of its glory only works on the 3.6 version of python and newer versions. It cannot be used on older versions of python and this can be a serious challenge for developers whose computers can only run older versions. The solution to this problem is simple: upgrade the python version if possible.
- Compiler Issues
The python compiler finds it challenging evaluating expressions within interpolated strings. It usually requires us to denote specifically what string we want it to evaluate. Also, when other prefixes such as “b”, “r”, or, “i” are used, the compiler may find it very difficult to correctly read the code at compile time thereby changing the meaning completely. This is a major problem with python string.format. To solve this problem all we need to do is place the letter “f” before the python center string. This does not only denote what we want the compiler to evaluate but it also helps the compiler read the code correctly as well as give the correct output.
Conclusion
There are many great things about the python programming language and python fstring is simply one of them. It makes coding more fun for developers and has many distinct features as we have seen above. If you haven’t started using it yet, you may want to give it a try right away. But first, check out our coding course to strengthen your foundational knowledge on all the basics of python.
Remember to also subscribe to our email newsletter so that you can be the first to know it whenever we drop new articles just like this one.