How to Write Your Own Pseudocode and Why You Need It.
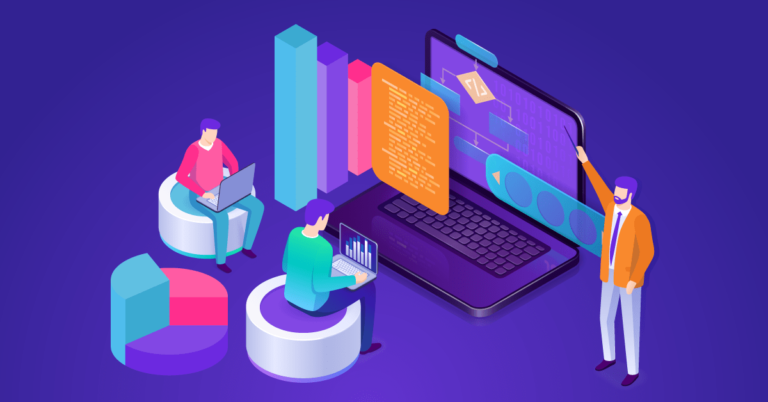
When it comes to writing computer codes, brainstorming is crucial. And you’ll probably agree that writing code for a new program isn’t easy. Well, you don’t have to jump straight into writing codes when you are assigned a huge project. You should come up with an outline of how you want to design the program before you start writing the code. This outline is referred to as a Pseudocode. And knowing how to write Pseudocode might be really useful.
Pseudocode is extremely useful and it is, by far, one of the best ways for programmers to represent an algorithm’s instinctive step. It ensures that no details or information about the algorithm are missed. Furthermore, it allows the programmer to focus on the algorithm rather than getting distracted by the coding process.
In this article, we are going to learn what Pseudocode is, how to write one, and read Pseudocode easily.
What is Pseudocode?
The Pseudocode is coined from the words Pseudo (false) and Code (coding). Pseudocode is not actually a computer programming language itself. It’s more of a representation of what you’re going to write using the English language or symbols. It is similar to skeleton algorithms.
Pseudocode is an informal way of analyzing programming that does not rely on strict programming language, syntax or underlying technology considerations. Pseudocode can be thought of as a way to create a program’s outline or rough draft. It is, nevertheless, a useful technique of summarizing the flow of a program without getting too involved with the actual coding.
Pseudocode frequently follows the structural rules of a standard programming language, but it is written for humans rather than machines. The goal of using Pseudocode is to make it easier for people to grasp than traditional programming language code, as well as to provide an efficient explanation of an algorithm’s main concepts.
Why should you learn Pseudocode?
Some of the many reasons why a programmer should understand Pseudocode are listed below.
- To explain the mechanics of a code.
If you’re working with a group of programmers, Pseudocode can help you explain how a program works. Unlike other programming languages, Pseudocode will be easy for others to read and understand. One advantage is that you won’t have to learn any other programming languages in order to explain the concept of your code. For instance, If you work with a python developer and are a C programmer, you don’t need to learn python to explain the algorithm’s concept. All you have to do is explain it in Pseudocode, and the other programmer will be able to implement it in Python if necessary. Moreover, having proper knowledge of Pseudocode will give knowledge of how to read Pseudocode of other programmers in your team.
- Ease up code construction.
Generally speaking, brainstorming can help you complete a task more quickly. The same can also be said about Pseudocode. It helps you complete tasks faster when writing new codes. Furthermore, it makes coding easy and effective without generating too many syntax errors.
A Pseudocode’s principal objective is to illustrate what each line of a program should perform. As a result, it speeds up the coding process and makes it easier to trace down problems. And to be able to quickly generate a simple way for resolving these coding errors.
Furthermore, you will only be able to write simple and effective lines of code using Pseudocode. You won’t need to add too much to your code because you already know what you want to do.
- Pseudocode is good for documentation.
As a data scientist, documentation might benefit you while working on a project that is similar to the one you have completed previously. In this case, Pseudocode can assist you. Pseudocode makes documentation easier because it is easier to read and understand, especially for nonprogrammers. Furthermore, Pseudocode might serve as an excellent starting point for your project. The Pseudocode is sometimes included as a docstring at the start of the code file.
- A good middle point for flowchart and code.
Many programmers use flowcharts to depict the program’s flow. Moving from a flowchart to coding, however, can be difficult for some. As a result, Pseudocode is the ideal approach for transitioning between stages, and it is even smoother than the formal. However, learn =ing Pseudocode and flowchart tutorials can present a great way to tackle programming problems.
What Pseudocode is not.
There are numerous advantages to learning Pseudocode and using it in your project. However, just as knowing what Pseudocode can do is crucial, knowing what it can’t is also important.
Here’s a list of things Pseudocode can’t perform.
- Pseudocode is a visual representation of your concept. This is due to the fact that your computer does not comprehend the code. It is solely available to programmers in order to understand the program’s flow and direction. More like a coded flowchart for your programs
- Unlike programming languages, there is no standard format for writing Pseudocode. As a result, it is only intended for you and your team to understand. The standard for writing Pseudocode differs from one person to another and from one company to another.
Pseudocode basic constructs
SEQUENCE, WHILE, IF-THEN-ELSE, REPEAT-UNTIL, FOR, and CASE are the six structured programming constructs represented by Pseudocode’s “structured” component. Each of these constructs has the ability to be embedded inside another construct. The logic, or flow of control, in an algorithm is represented by these constructs.
However, it has been demonstrated that every “good” algorithm can be implemented using only the first three basic components for the flow of control. Although these constructs are sufficient, the other three constructs are often useful.
- SEQUENCE
- WHILE
- IF-THEN-ELSE
- REPEAT-UNTIL
- CASE
- FOR
Pseudocode examples
- SEQUENCE
Writing one action after another, each on its own line, and all actions aligned with the same indent, indicates sequential control. The activities are carried out in the order in which they are written (from top to bottom).
Example.
READ width of the square
READ height of the square
COMPUTE area as width times height
Other Action Keywords
To denote common input, output, and processing actions, a number of terms are frequently used. These keywords include
Input: READ, GET, OBTAIN
Output: PRINT, SHOW, DISPLAY
Compute: COMPUTE, DETERMINE, CALCULATE
Initialize: SET, INIT
Add one: INCREMENT, BUMP
- WHILE
The WHILE constructs specify a loop with a test at the top. The terms WHILE and ENDWHILE mark the start and end of the loop, respectively. The general format is as follows:
WHILE condition
sequence
ENDWHILE
Example
WHILE total student < teacher
Compute Attendance as Students + teacher – parent
ENDWHILE
- IF-THEN-ELSE
Example
IF the total number is greater than 1000 THEN
display all numbers
ELSE
ignore all numbers
ENDIF
It’s worth noting that ELSE can be used in situations when the condition is either true or false. If the condition is met, the first sequence will be run; otherwise, the second sequence will be run.
- REPEAT-UNTIL
The REPEAT-UNTIL loop is similar to the WHILE loop, with the exception that the test is run at the bottom of the loop rather than at the top. REPEAT and UNTIL are two keywords that are utilized. The general format is as follows:
REPEAT
sequence
UNTIL condition
- CASE
CASE, OF, OTHERS, and ENDCASE are four keywords and conditions that are used to indicate the various alternatives.
Example
CASE Title OF
Mr : Print “Mister”
Mrs : Print “Missus”
Miss : Print “Miss”
Ms : Print “Mizz”
Dr : Print “Doctor”
ENDCASE
- FOR
FOR is often called a “counting” loop.
Example
FOR iteration bounds
sequence
ENDFOR
When writing Pseudocode, these six constructs are primarily used. You may, however, add more constructs (keywords) to your Pseudocode depending on the field you work in. As long as you never use these keywords as variable names and they are well recognized by others in your team.
Rules of writing Pseudocode
Here are some Pseudo code rules to follow for maximum efficiency.
- Always capitalize the basic constructs because they indicate what should be done. “READ,” “OBTAIN,” and “GET,” for example.
- It’s critical to use only one statement per line for smooth reading and comprehension.
- Concentrate on the algorithm rather than the core coding. Instead of focusing on how to code the program, just emphasize what you want it to perform.
- To arrange related activities together, use indentation (just like in any other programming language). It will make for an easier read.
- Use any of the END keywords to end multi-line sections (ENDIF, ENDWHILE).
More tips on how to write Pseudocode algorithm
There are several fundamentals to learning how to write Pseudocode. When you want to write an algorithm in Pseudocode, these Pseudocode tips will help you.
- Understand the uses
If you don’t understand how to use Pseudocode, it will be quite difficult for you to learn how to use it. It’s also crucial that you understand how it’s used in programming.
- Pseudocode is subjective
Pseudocode, as previously mentioned, is subjective. In other words, there is no standard for writing Pseudocode. However, Always keep in mind that the purpose of writing a Pseudocode is to write down all that is on your mind in a clear and straightforward manner.
- Follow standard pseudo code rules and constructs
It’s possible that there isn’t a standard technique to write Pseudocode. There are, however, pseudo code rules and constructs that will ensure that everyone with whom you collaborate understands what you’re stating.
For effective Pseudocode, stick to the core constructs and standard guidelines outlined above.
- Keep it simple and detailed
Any programmer should be able to understand a Pseudocode if it is straightforward and detailed. It should be easy to read and understand. When necessary, add comments to illustrate essential processes. Remember that you’re writing what the project will do, so summarizing the Pseudocode isn’t a good idea.
- Review the Pseudocode before coding
Before using Pseudocode in programming, review the Pseudocode to ensure that any flaws and mistakes have been eliminated. This will relieve you of the worry of having to reprogram if a mistake occurs during the pseudo coding process.
Final thought
The main aim of Pseudocode is to identify how you want your program to work before you start coding it. It’s critical to write the best possible Pseudocode that answers all of the project’s issues and points it in a clear direction. And with this Pseudocode tutorial, you will have the skills needed to write Pseudocode like an expert.
Nevertheless, you still have a need to be a part of Data Science Club, SDS Club, where you can learn up-to-date Data Science hacks, take well-structured courses, and network with fellow data scientists at all skill levels.
Was this article useful?
Subscribe to our newsletter for timely updates about new posts. Also, share this insight with your network on social media today!