Pandas vs Numpy: The Decisive Guide
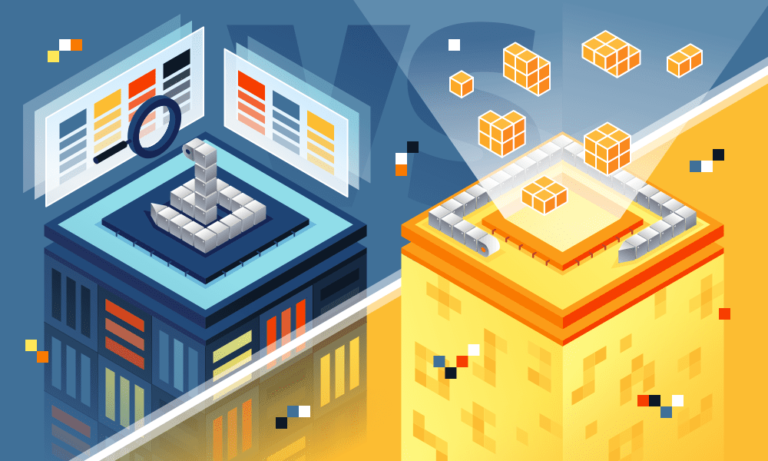
Python usage is continuously increasing. With wide applications in several areas including machine learning and scientific computations. And for this reason, more and more libraries and packages are being built and released so that developers can find it a lot easier to cod+e and build applications and programs. Pandas and NumPy are two very useful libraries that python has developed to make coding easier. And today, in this article, we will be comparing NumPy vs Pandas to see how they differ. But before we dive into the comparisons, let us understand what they are.
What is Pandas
Pandas were coined from Panel Data (an Econometric derived from Multidimensional data) and first came into existence in 2008 after it was developed by Wes McKinney. By definition, Pandas python is an open-source library that allows developers to run high-performance data manipulation as well as data analysis.
On its own, Pandas is already an excellent tool. But to reach the highest possible peak and become an extraordinary tool, it has to be combined with NumPy. The NumPy libraries contain all that is required to initialize and operate all Pandas objects.
Irrespective of the source of the data that you throw at it, there are essentially five steps that Pandas takes to achieve this high-performance data analysis and they include; loading, manipulating, preparing, modeling, and analyzing.
What is NumPy
NumPy is short for ‘Numeric Python.’ It is also a python library built on C language. It was created in 2005 by Travis Oliphant. NumPy was created to serve as an extension module of Python. By definition (and just as the name implies), it is a Python package that can be used to perform different forms of numerical computations as well as to process both singular-dimensional and multi-dimensional array elements.
Alone, NumPy can handle a large amount of data pretty well and it can display a level of computation and calculation that is considered to be way faster than the regular Python array. But when combined with Pandas, these capabilities become almost limitless.
Aside from data handling, NumPy can also be correctly applied in other types of applications such as data reshaping as well as matrix multiplication.
How Are They Used
Pandas is heavily dependent on NumPy because all the functionality Pandas requires to work properly is usually contained within the NumPy library. The implementation of all Pandas data objects and elements alone is based on NumPy and then there is the fact that both libraries share the same features. For these reasons, they can hardly be used separately. This answers the question ‘should I use Pandas or NumPy?’ just in case anyone is asking.
So, what is the use of NumPy and Pandas in Python? Collectively, both packages are useful in a part of Python known as the Scientific Python stack. That is, they are used in scientific computations. SciPy is what developers use as the short form for Scientific Python and is an umbrella of libraries and tools that function effectively in performing all kinds of specialized scientific calculations, manipulations, and analysis. It is believed that if an operation has anything to do with scientific computing then it is most likely implementable on SciPy.
NumPy is useful in the following types of operations:
- Integrating and computing C and C++
- Performing linear algebra
- Performing random number capability
- Multi-dimensional and matrix manipulations
- Vectorizations and generic data analysis
Pandas on the other hand can be used in the following types of operations:
- Time and non-time series data structures
- Performing mathematical operations on data structures
- Performing relational operations
- Handling missing data
- Performing arbitrary matrix data involving rows and columns
We can also have a deeper understanding of how these libraries are used by seeing the core Python operations that depend entirely on these libraries. For instance, let’s look at some operations that depend on the Python NumPy:
- ndim:
This is an operation that allows us to find the dimension of an array. Be it a single-dimensional array or a multi-dimensional array
- itemsize:
This operation allows us to find the exact size of an object or element in byte
- dtype:
This operation can be used to find out what data type the elements stored in an array are
- reshape:
This operation allows us to make some changes to the rows and columns in an array to view the objects differently
- Slicing:
The slicing operation allows us to extract particular sets of an element from an array
- linspace:
This is an operation that returns evenly spaced numbers over specified intervals
- max/min:
The max/min operation allows us to find the maximum, minimum as well as sum values of an array
- Square Root and Standard Deviation:
This operation helps find the square root as well as the standard deviation of the numbers contained in an array
- addition:
We can also perform the addition of the values contained in an array. But addition is not the only operation possible with NumPy. We can perform subtractions, multiplications, and divisions as well
- Stacking:
It is also possible to concatenate two arrays by stacking them both vertically and horizontally rather than adding them
- ravel
This operation allows us to convert one NumPy array into a single column or convert Pandas series to NumPy array
What Is The Difference Between NumPy vs Pandas?
Before we compare NumPy vs Pandas, let us once again establish some facts; using Pandas requires making provisions for NumPy as well because Pandas and its series are dependent on the functionalities made available by NumPy array. For instance, you have to convert Pandas DataFrame to NumPy array before you can perform certain operations.
However, even though both libraries are always used together, it is possible to point more than a difference between NumPy and Pandas as they are both unique in their ways. Below, we, therefore, highlight the difference between Pandas and NumPy:
- Type of Data
NumPy especially works with numerical and mathematical data while Pandas works with tabular data
- Available Tools
Another difference between Pandas vs NumPy is the type of tools available for use in both libraries. With Pandas, we can use both Pandas series and Pandas DataFrame, whereas in NumPy we use the array tool
- Speed and Memory Usage
NumPy is faster and consumes less computation memory when compared with Pandas
- Type of Object Used
The type of objects used is another way to differentiate Python Pandas vs NumPy. While Pandas uses the object of DataFrame in a 2d table format, NumPy uses the multi-dimensional array
What is Pandas Series and NumPy Array?
A Pandas series is a type of list also referred to as a single-dimensional array capable of taking and holding various kinds of data including integers, strings, floats, as well as other Python objects. The array can be labeled in which case the labels can be called index. To help you understand this better, think of a Pandas series as a column on an excel spreadsheet.
A collection of Pandas series is what is generally known as a Pandas DataFrame. And because a DataFrame has several columns it is possible to add a NumPy array to Pandas DataFrame.
We can convert NumPy array to Pandas series or simply create a Pandas series from a NumPy array using a piece of code like the one below:
# import pandas as pd
import pandas as pd
# import numpy as np
import numpy as np
# simple array
data = np.array(['g','e','e','k',’s’])
ser = pd.Series(data)
print(ser)
The output would be:
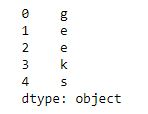
NumPy array is an effective N-dimensional array of objects that comes in rows and columns. The arrays are usually arranged in memory as continuous blocks that are mostly of the same type and size. This is usually what is responsible for the incredible speed associated with NumPy.
The array can either be a vector or a matric. A vector array is usually a single-dimensional array, while matrix arrays are 2-dimensional. As a rule, NumPy arrays are always homogenous containing only one data type or size.
There are other functions allowed by this tool apart from the many numerical computations we can perform with it. These operations, which we have seen earlier include; logical operations, selection of elements, reshaping, slicing, stacking, as well as splitting. Hence, whenever we want to run high-performance operations we can simply convert Pandas to NumPy or convert Pandas DataFrame to NumPy array.
Some Examples of NumPy vs Pandas Working in Python
We will now look at some examples of how NumPy array vs Pandas DataFrame is used separately as well as jointly.
Below is how we can use NumPy array to calculate the cosine of some numbers:
import numpy as np
X = np.random.random((4, 2)) # create random 4x2 array
y = np.cos(X) # take the cosine on each entry of X
print y
print "\n The dimension of y is", y.shape
And the output would be:
[[ 0.95819067 0.60474588]
[ 0.78863282 0.95135038]
[ 0.82418621 0.93289855]
[ 0.67706351 0.83420891]]
The dimension of y is (4, 2)
Using it separately, we can use Pandas DataFrame to find out the electoral votes of some states in the US:
import pandas as pd
# create data
states = ['Texas', 'Rhode Island', 'Nebraska'] # string
population = [27.86E6, 1.06E6, 1.91E6] # float
electoral_votes = [38, 3, 5] # integer
is_west_of_MS = [True, False, True] # Boolean
# create and display DataFrame
headers = ('State', 'Population', 'Electoral Votes', 'West of Mississippi')
data = (states, population, electoral_votes, is_west_of_MS)
data_dict = dict(zip(headers, data))
df1 = pd.DataFrame(data_dict)
df1
For the above code, the output would be:
Electoral Votes | Population | State | West of Mississippi | |
0 | 38 | 27860000.0 | Texas | True |
1 | 3 | 1060000.0 | Rhode Island | False |
2 | 5 | 1910000.0 | Nebraska | True |
When we decide to combine them, we can do even more. For instance, we may decide that we want to know what is the mean value of the population above. For that, we will run the code:
print df1['Population'].mean()
And get the output:
10276666.6667
Conclusion
Because they are originally separate libraries, it is expected that we will see some differences when comparing NumPy vs Pandas. However, these differences are few and almost inconsequential as both packages are designed to function together. That is, Pandas is largely dependent on NumPy for its functionalities while NumPy is dependent on Pandas for both extension and expansion. Together, they make a very powerful weapon in the hands of any developer.
Remember to subscribe to our email newsletter so you can always know when we drop another interesting article like this one and don’t forget to share this knowledge with your friends.
Leave a comment