The Practical Guide to Tree Traversal Algorithms
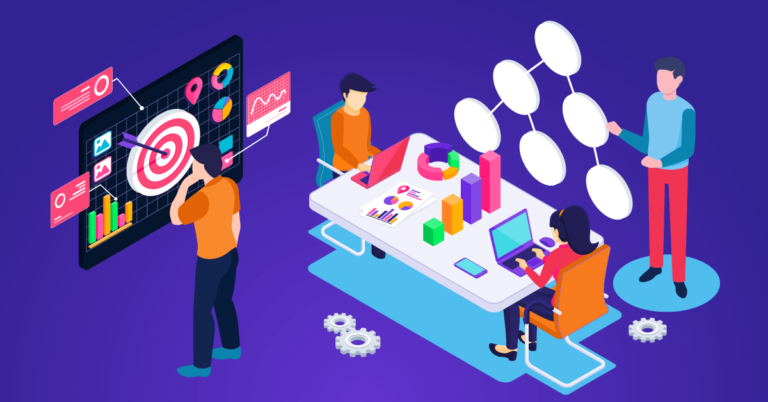
Tree traversal algorithms work simply by visitation. Just as you would visit your friends and families, check up on them, and update them about the happenings in your life, tree traversal algorithms work the same way. The only difference is that the visitation is a data structure in the form of a graph that looks like a tree with leaves called nodes.
Looking to learn how the whole tree traversal complexity works in simple and concise explanations? This guide is for you. As developers ourselves, we have put together this guide to help you understand how the whole concept of tree traversals functions. We’ll explain this to you in words that are both relative and simplifying.
This guide will look at the fundamentals of tree traversal algorithms — nature, meanings, and application. It will detail the types of tree traversal algorithms there are as well as the different techniques that are used in traversing. It will explain the recursion and iteration of traversing nodes or trees to achieve your printed stack output.
What does Tree Traversal mean?
Tree traversal is the scientific or algorithmic process of visiting all the nodes in the traversal of a binary tree to achieve a printed output. Four key terms made up this definition: algorithm, nodes, binary tree, and output. We’ll look at each one of them.
Algorithm
The traversing of trees can be recursive or iterative and this is what makes it an algorithmic process. Algorithms are a series of well-defined computer instructions that solve a problem or enact a computation.
In Data Science, of which Machine Learning is a subset, machines can be trained to iterate trees and nodes to achieve a specific result. Such an iteration, however, will require some algorithms.
Nodes
What are nodes in tree traversal? Think of nodes as a parent with no child, one child, or two children. You may also think of them as a lineage with no descendant, one descendant, or two descendants. Whether you think of them as children or descendants, know that they can never go beyond two in binary trees.
In theory, nodes are structures, connected via edges or lines or links, that contain values or represent certain conditions, or even highlight other data structures. Nodes make up a tree in Data Science and they have zero or one or two child nodes.
Binary Trees
Trees are otherwise known as data structures. A single tree contains several nodes that are interlinked. Trees begin with a parent node that is drawn to pave the way for other nodes. These other nodes, when looked at, give the perception of being children to the parent node.
A simple binary tree traversal example that captures what the trees look like is the common family tree. As family trees are drawn, the same way binary trees are drawn. What makes binary trees binary is the 2 children no single element in the tree must exceed. All elements are confined to no child, one child, and, at most, two children.
Printed Output
The printed output is the result of all nodes and the values contained in them. You can print binary output into C++, C, Java, Python, C#, JavaScript, and several other Machine Learning languages. You can code printed output from scratch or even use software frameworks.
Iteration is never achieved until the derived output is meaningful to Data Engineers and Scientists. By being meaningful, we mean the output must be printed in programming languages to better help Data Scientists understand the content in a tree structure.
What Are the Tree Traversal Techniques?
A technique is an operation performed on a desired object or subject. Tree traversal techniques are operations used in traversing trees and dissecting the values of their nodes. But how do Data Scientists go about doing this?
Engineers and scientists deploy two major tree traversal methods in deconstructing the values contained in binary trees. These two techniques are depth-first traversal and breadth-first traversal. We’ll look at each of these tree traversal methods.
Depth-first Traversal
Also known as depth-first search (DFS), the technique involves concentrating on the placement of the root node or the parent node (whether first, or second, or third in order). After placing, the technique involves exploring all possible nodes along the branch before retracing movement to the root node wherever it is placed. There are three ways DFS can be done: Preorder, Inorder, and Postorder.
Preorder DFS Traversal
Preorder contextually stands for before order. That said, depth-first traversal through Preorder can be achieved by starting with the Root Node first before moving to other nodes. The Root Node comes first in order before moving to the Left Node then to the Right Node.
For symbolism, to traverse binary trees through Preorder is NLR (Node, Left, and Right). The node is the primary node — parent and the root node — while others are secondary nodes. Here is instruction or algorithm to follow if you still don’t understand how the preorder traversal works:
- Visit the Parent (Root) Node;
- Then visit the Left Child Node;
- End by visiting the Right Child Node.
Inorder DFS Traversal
Inorder traversal is a bit different from preorder. Also, as the term implies, it has to do with starting with the left child node first before the parent node and then concluding with the right child node. Here you will notice that the Root Node is in order, between the left child node and the right child node.
For symbolism, to traverse binary trees through Inorder is LNR (Left, Node, and Right). “Left” is the left child node, followed by the Root Node, and ended by “Right” which is the right child node. Here is yet another algorithm to understand how Inorder works.
- Visit the Left Child Node first;
- Then visit the Parent (Root) Node;
- End your visits with the Right Child Node.
Postorder DFS Traversal
Postorder likewise implies what it stands for. It works by operating on the left child node first and then the right child node before finalizing the binary trip with the root node. Concentrating on the root node after exploring other nodes makes this technique Postorder.
For symbolism, traversing nodes using Postorder is LRN (Left, Right, and Node). The “Left” as you already know is the left child node, the “Right” is the right child node, and “Node” is the parent node. Operate Post Order by:
- Visiting the Left Child Node first;
- Following with the Right Child Node;
- Bidding your farewell on the Parent Node.
Breadth-first Traversal
Also known as level-order tree traversal (LOTT) or Breadth-first Search (BFS), this technique eats up all the nodes by procedures or levels.
For example, if you’re faced with two binary trees and these trees are presented to you in different levels, and you are to use the level-order traversal technique, you first traverse all the nodes at the first level before moving to other levels. This is what makes this technique level-order.
Breadth-first traversal can be done using either recursive tree traversal or iterative tree traversal. Tree traversal recursion is a form of solving binary nodes by working around the smaller sub-nodes (children) exactly once before the parent node. Iteration is executed by repeating the nodes until the desired result is achieved.
Rather than just visit nodes as in depth-first traversal, breadth-first is more concerned about exploration. This means you cannot visit the nodes without exploring all adjacent nodes. This is what makes it breadth-first.
Tree Traversal Structure and Uses
Nodes are not the same; they are unique from one to another. The uniqueness should be familiar alongside the uses of the different tree traversal methods. Finally, the application of the techniques should be looked at and not just theorized.
Structure of the Nodes in BST
Binary search trees (BST) consist of tree nodes that are differently structured. A node in BST can be
- Full
- Complete
- And Perfect.
A node is said to be full when it has no children or only two children. It is complete when it can be linked from top to bottom, and from left to right. And it is perfect when all the nodes have two children that are on the same level.
Uses of Tree Traversal Techniques
The following are some of the ways tree traversal techniques can be used:
- Preorder traversal can be deployed if a developer ever needs to make a polish notation from expression trees.
- Inorder traversal without recursion is the most used technique and this is because it returns values from expression in the exact order.
- Postorder traversal allows developers to initiate a reverse Polish notation from expression trees.
Conclusion
Understanding how to traverse a tree by visiting and exploring nodes in tree traversal algorithms is essential to dissecting your tree and graph structure. You can either iterate your binary trees or execute them by recursion. You can visit them depth-first or explore them breadth-first.
As interesting as this sounds, understanding it relies on knowledge. Are you looking to learn or polish your Data Science skills or any other specific aspects of it?
Data Science is not impossible. We’ve been there. We know how it works.
It’s very easy. We offer unique online courses with easy and comprehensive Data Science roadmaps.
Our courses contain several career-boosting features topped with discounts and special mouthwatering offers that are hard to ignore. Check them out today!
Subscribe to our newsletter now to stay up to date on new posts, discount offers, and latest DS tricks. Also, don’t forget to share this article with your network.
Leave a comment