Troubleshooting: The Ultimate Tutorial on Python Error Types and Exceptions
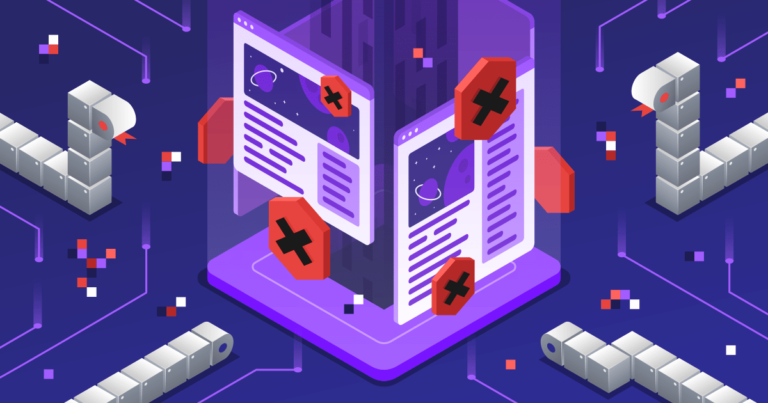
Errors and exceptions play a crucial role in a program’s workflow. Although Python errors and exceptions may sound similar, there are key differences between them that make the whole error handling process of a program much more complicated.
As developers, we need to ensure that the programs we write can properly handle as many Python exception types as possible and are not affected by any of these disruptions. However, before we learn how to do that, it is essential that we learn about these errors and exceptions well enough to make our programs foolproof.
Python Types of Errors
The types of errors Python programs may throw, can occur prior to its execution as well as during runtime. They are also potential indications of fatal problems that might be in the program.
The exceptions, which are also known as runtime errors, can only be identified during the program’s runtime. Once thrown, these exceptions may disrupt the intended flow of the program and may cause a crash. Then, there are errors that may or may not be identified prior to its runtime. However, it is vital that they should not be handled by catching as opposed to exceptions. This is mainly due to the unrecoverable nature of errors. The correct practice is to handle them at the time of the writing of the code.
In this tutorial, we will be mainly talking about the major three types of errors SyntaxError, RecursionError, and OutofMemoryError.
Python Types of Exceptions
Exceptions in Python always occur during runtime, and if they are handled well, the end-users may even never notice any problematic nature with your program. The issue with the exception is that unlike errors, they are difficult to be anticipated. However, identifying and handling them in advance can prevent potential crashes, failures and facilitates smooth execution.
According to the exception hierarchy of Python 2, we can identify the three main types of Python exceptions, such as StopIteration, StandardError, and Warning. Although in Python 3, the hierarchy has been redefined by removing the StandardError type and taking many of the Python multiple types of exceptions altogether, we will follow the older Python exception hierarchy for the ease of understanding when we are talking about exceptions. It should also be noted that the functionality of these exceptions has not been changed, but only the parent Python exception types have been changed.
1. Types of Errors in Python
We now have an idea about the error types Python has. In this section, we will go over these types of Python errors one by one.
a. Python Error Types: SyntaxError
Syntax errors occur due to a line/ multiple lines of code being deviated from the expected syntax. The parser detects them and, therefore, can also be called parsing errors.
We will now look at an example to get a better idea about it. In the following code, we have written a print statement following Python 2 style syntax. Once we execute it, it will notify us with a SyntaxError.

Output:

Let’s look at another syntactic error.

In the above code, we have written the body inside the if statement without proper indentation. Therefore, this will output an IndentationError.
Output:

These errors are detected prior to execution, and therefore, if we can execute our code initially without this error, we are good to go for the rest of the execution.
Another
b. Python Error Types: RecursionError
Recursion errors are related to the stack as one can execute one method inside itself only so much without running out of the limitations imposed by the stack.
We will now define a recursive function and call it, triggering an infinite recursive loop. In other words, it will call itself nonstop until it has reached the stack limitations.

Output:
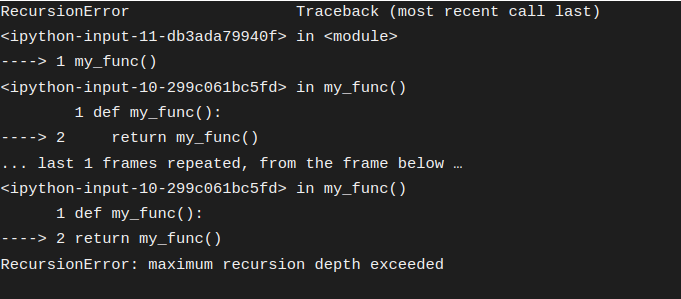
c. Python Error Types: MemoryError
All the variables and other objects of programs we write and execute are being stored in the RAM of your device. Let’s say we have written a data cleaning algorithm, and we need to check it with a big data file. However, if this file is a very large object that cannot be handled by our RAM, we will be alerted with an MemoryError, and the program will stop to recover.
Now that we have covered Python error types let’s move on to Python exception error types.
2. Python Exception Types: StopIteration
This exception is raised in the event where there are no more items or objects produced by an iterator. It is generally raised by the __next__() function.
We will go ahead and create a class to generate consequent numbers and print them. For ease, we will only print numbers 0 to 5.
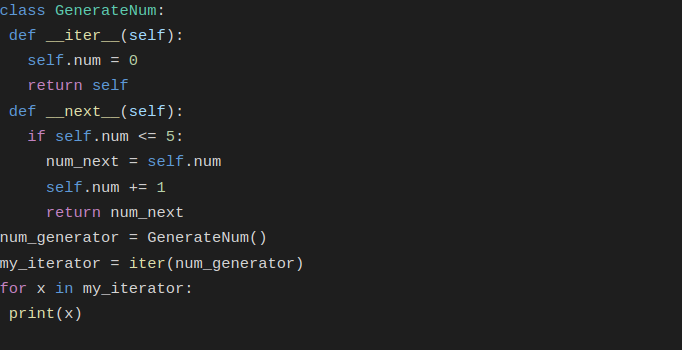
This would output
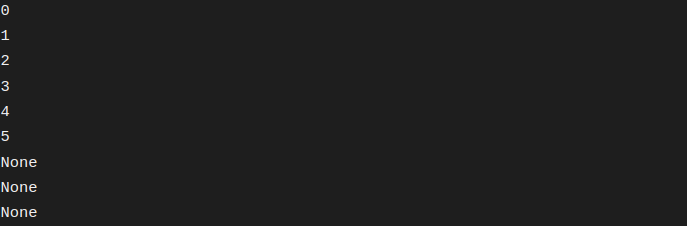
This would keep printing ‘None’ infinitely. We can easily solve this by raising a StopIteration exception. Let’s modify the __next__ function as follows.
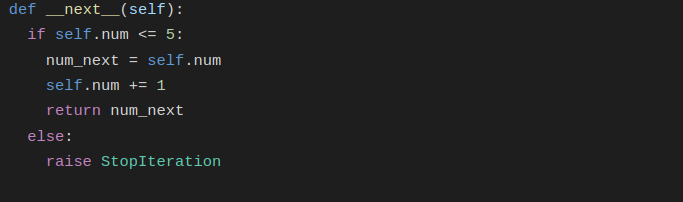
When we execute the modified code, it will only print numbers from 0 to 5 and stop the execution.
3. Python Exception Types: StandardError
In Python 2 exception hierarchy, the StandardError exception is the base class for many of the common exceptions that may arise when the program is being executed. We will go over a few of them now.
a. ArithmeticError
This Python exception type is raised for arithmetic related errors. This base class has the exceptions OverflowError, FloatingPointError, and ZeroDivisionError.
Let’s look at an example. We will attempt to divide a number by zero.

Output:
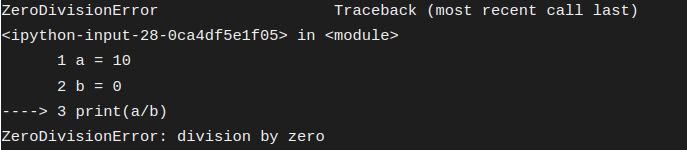
b. AssertionError
Assert statements are used by developers in debugging the code to test conditions. If the assert statement succeeds, the program flow is not disrupted. However, if the statement fails, then an AssertionError is raised.
Let’s look at an example.

Output:
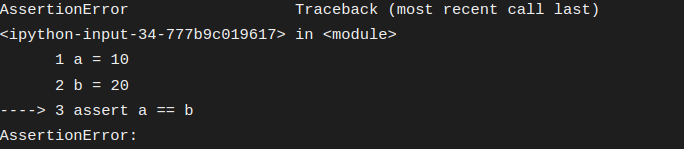
c. AttributeError
This exception is raised when an attribute assignment or a reference fails.
We will try to define an integer object and append another value to it. Since integer objects do not support appending, it will raise an AttributeError.

Output:
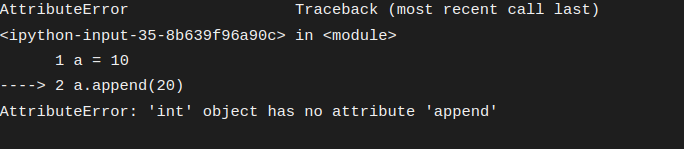
d. ImportError
This is the base class for ModuleNotFoundError exception, which is raised when a module that does not exist is imported.

Output:

e. LookupError
This is the base class for IndexError and KeyError, which are raised respectively when the index or the key is not invalid for mapping or sequence.

Output
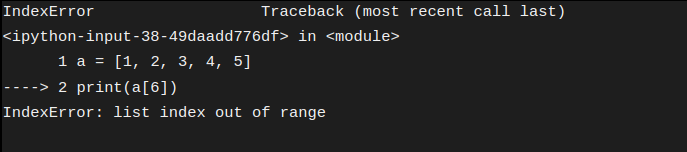
f. TypeError
This exception is raised when an inappropriate operation is applied to an object of another type.

Output:
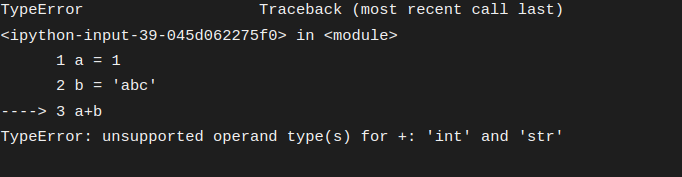
Although we only did discuss a few exception types Python has, there are many more exception types in the Python language. As a developer, one should be intuitive about learning more about the in-depth information with more developments being done, and codes are written. We will now move on to the next exception type, Warning.
4. Python Exception Types: Warning
Warning is the base class for categories such as UserWarning, PendingDeprecationWarning, DeprecationWarning, SyntaxWarning, RuntimeWarning, FutureWarning, ImportWarning, UnicodeWarning, BytesWarning, and ResourceWarning. As Warning base class is not the main focus of this tutorial, we will not be discussing this any further. However, it is helpful to remember that warnings do not disrupt the flow of our program but merely alerts us about any phenomenon of concern.
5. Handle Errors and Exceptions
Now that we are knowledgeable about what kind of exceptions we may come across, we are prepared to handle exceptions. The basic procedure to deal with exceptions starts with catching them and deciding what has to be done next. Moreover, the most fundamental idea in handling exceptions is ‘try’, ‘except’, ‘else’, and ‘finally’ blocks.
try – Contains the code that might throw an exception
except – What to do if there is an exception
else – If there are no exceptions, run this block
finally – Run this code despite whether there is an exception or not
Let’s look at some scenarios of catching exceptions using just try and except blocks.
a. Catching All Exceptions
Imagine that our program is not very complex. However, we do have one or two potential lines of code that may throw exceptions. Moreover, we are not concerned about what type of exceptions are thrown and only want to provide the program with a smoother flow. That is when we can use plain try and except blocks as follows.
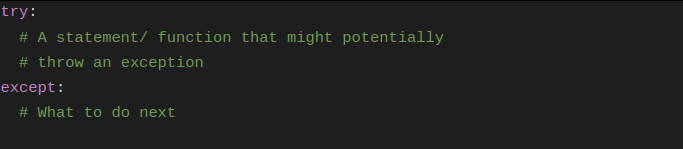
b. Avoid Catching Special Exceptions
Imagine we want to catch all exceptions except special ones such as SystemExit, KeyboardInterrupt, and GeneratorExit. We may want to proceed as follows.
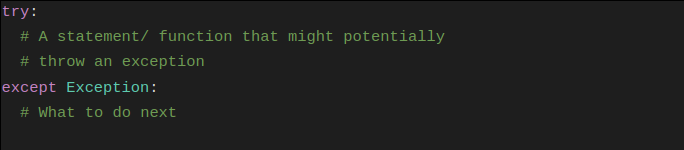
c. Catching Individual Exceptions
If we are to catch exceptions individually and handle them accordingly, we can proceed as follows.
We will try to catch an ArithmeticError here.

Output:

d. Using ‘else’ and ‘finally’ Blocks
Consider the following code.
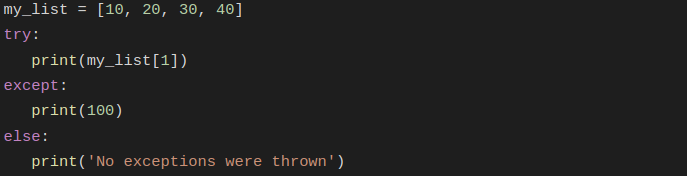
The above will produce the following output.

There were no exceptions thrown, and therefore, the code inside the else statement was executed.
We will now add a finally statement to the above code.
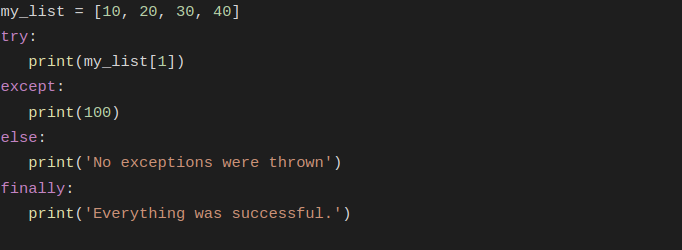
This will output,

So, there it is. That is how we can handle exceptions in Python.
Conclusion
Exceptions are an essential feature of Python to identify and address any issues and problems that may occur during the runtime. They can be considered a part of the core workflow of the program itself. Moreover, the flow of the program can be made much smoother and foolproof with the correct handling of Python errors and Python exceptions. If you are still starting with Python and require a better foundation before you move on to exception handling, this Python A-Z course or Python Beginner to Pro course might be the best one for you.