Your Guide to Different Ways of Initializing a Vector in C++
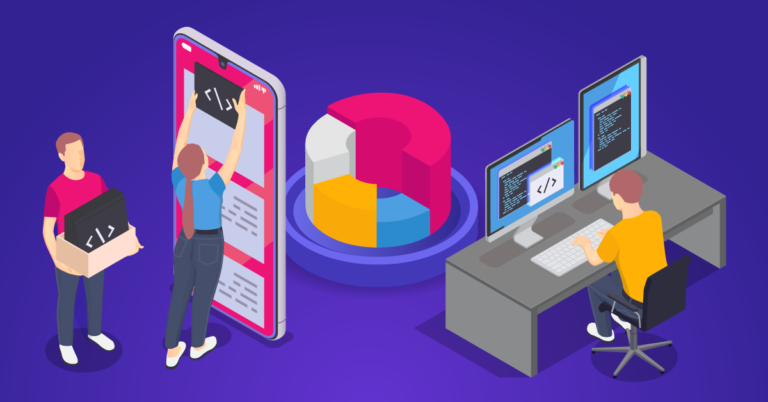
<img src=”http://gen.sendtric.com/countdown/7mrd8y5i38″ style=”display: block;” />
Are you a beginner just starting your C++ journey and you are finding vector initialization a bit difficult or you are looking for ways to go about it? Well, you don’t need to search further because this article is just the answer you need to your questions.
In this article, you will get to know how to initialize vector in C++, how to initialize vector vectors, if you need to initialize vectors in C++, c++ vector, c initialize vector in constructor, c++ vector example, how to initialize 2d vector in c++, how to initialize empty vector in c++ and so on. Sit back and have a nice time going through the guide.
How do you initialize a vector in C++?
C++ Vector
Generally in computer science and its related fields, vectors are STL containers used in storing data dynamically. They are referred to as the representation of arrays with properties of being able to increase or decrease in size depending on how elements are inserted or deleted. Vectors store data on a single entity on a single index which is synonymous with the way its static counterpart stores data. Insertion and deletion occur from the last index to the first index. In deletion, constant time is required but inserting an element takes differential time. This is because a vector needs to resize itself after an element is added.
Just the same way STL containers provide different functions, vectors also do the same. Here are three subcategories of part of the functions.
- Capacity: Capacity is a member function of the c++ vector that deals with the size and capacity of a vector container. Below are the types of capacity C++ vector provides.
reserve(), resize(n), capacity(), max_size(), empty(), size() and shrink_to_fit(), |
- Iterators: These are the types of functions that enable you to move through a vector container or iterate. Here are some of the iterators provided by a C++ vector.
rbegin(), rend(), cbegin(), cend(), begin(), crbegin(), crend(), and end() |
- Modifiers: This type of function is involved in the modification of vector containers. Examples of modifications that can occur include element removal, deletion, etc. Here are the types of modifiers vectors provide.
emplace(), clear(), assign(), pop_back(), swap(), push_back(), emplace_back(), and erase(), |
How to Create a Vector in C++
Vectors in C++ work by vector declaration in c++ that uses them. Commonly, the syntax of how to create a new vector in c++ looks like this
vector <type> variable (elements) |
A simple breakdown.
- Type: it defines a data type that is stored (e.g <int> <double> or <string>)
- Variable: this is the name chosen for the date
- Elements: Specify the number of elements for the data.
C++ Vector Example
The query below is a typical type of program used to check if the vector contains a duplicate element. If the vector contains a duplicate element, it returns true but if not, it returns false.
#include<bits/stdc++.h> using namespace std; //function to check if the vector //contains a duplicate element or not bool containsDuplicate(vector<int>& nums) { //check if the vector is empty if(nums.empty()) return false; //sort the vector sort(nums.begin(), nums.end()); int i = 0; int j = i+1; while(j<nums.size()) { //check if the current element //is not equal to its successor if(nums[i] != nums[j]) { i++; j++; } else return true; } return false; } int main() { vector<int> nums{ 2, 3, 5, 1, 2, 4 }; //print vector elements cout <<“Vector elements are “; for (int x : nums) cout << x << ” “; cout <<endl; //print result if(containsDuplicate(nums)) cout <<“Vector contains a duplicate element”; else cout <<“Vector does not contain a duplicate element”; } |
Ways to Initialize A Vector in C++
In vector initialization, vectors can be initialized without defining the size of the container because the size dynamically increases or decreases. To initialize a vector, there are six different ways on how to initialize a vector in c++.
- Using an existing array
The use of an existing array in vector initialization is one of the most standard ways. A vector can be initialized by using an already defined array. Doing this involves passing the elements of the array to the iterator constructor of the vector class.
Illustration
// CPP program to initialize a vector from // an array. #include <bits/stdc++.h> using namespace std; int main() { int arr[] = { 10, 20, 30 }; int n = sizeof(arr) / sizeof(arr[0]); vector<int> vect(arr, arr + n); for (int x : vect) cout << x << ” “; return 0; } Output: 10 20 30 |
- Using the overloaded constructor of the vector class
In the overloaded constructor, the constructor accepts two elements. The size of the vector and the value to be inserted is provided during the vector initialization c++. The value provided will be inserted into the vector multiple times which is equal to the provided size of the vector. Below is an illustration of how to go about it.
Illustration
Input: vector<int> v1(5, 2)
Output: The resulting vector will be:
2 2 2 2 2
Input: vector<int> v2(4, 1)
Output: The resulting vector will be:
1 1 1 1
#include <iostream> #include <vector> using namespace std; int main() { // initialize size int size = 5; // initialize vector using an overloaded constructor vector<int> v1(size, 2); // print the vector elements cout << “The vector v1 is: \n”; for (int i = 0; i < v1.size(); i++) { cout << v1[i] << ” “; } cout << “\n”; // initialize vector v2 vector<int> v2(4, 1); // print elements of vector v2 cout << “\nThe vector v2 is: \n”; for (int i = 0; i < v2.size(); i++) { cout << v2[i] << ” “; } cout << “\n\n”; return 0; } |
- Using an existing vector
This involves passing the iterator of an already initialized vector to a vector class constructor. Doing this leads to the initialization and population of a new vector by the elements in the old vector. Below is an illustration of the code.
Illustration
// CPP program to initialize a vector from // another vector. #include <bits/stdc++.h> using namespace std; int main() { vector<int> vect1{ 10, 20, 30 }; vector<int> vect2(vect1.begin(), vect1.end()); for (int x : vect2) cout << x << ” “; return 0; } Output: 10 20 30 |
- Passing an array to the vector constructor
This is another important method to initialize vectors. It involves passing an array of elements to the vector class constructor. Here, array elements will be inserted into the vector in the same order leading to the automatic adjustment of the size of the vector.
Illustration
Input: vector<int> v1{10, 20, 30, 40, 50} Output: The resulting vector will be: 10 20 30 40 50 Input: vector<char> v2{‘a’, ‘b’, ‘c’, ‘d’, ‘e’} Output: The resulting vector will be: a b c d e #include <iostream> #include <vector> using namespace std; int main() { // pass an array of integers to initialize the vector vector<int> v1{10, 20, 30, 40, 50}; // print vector elements cout << “The vector elements are: \n”; for (int i = 0; i < v1.size(); i++) { cout << v1[i] << ” “; } cout << “\n\n”; // pass an array of characters to initialize the vector vector<char> v2{‘a’, ‘b’, ‘c’, ‘d’, ‘e’}; // print vector elements cout << “The vector elements are: \n”; for (int i = 0; i < v2.size(); i++) { cout << v2[i] << ” “; } cout << “\n\n”; return 0; } |
- Using the push_back() method to push values into the vector
You can use this method to insert or push elements into a vector. Each element is inserted at the end of the vector and the vector size also increases by 1. The name of the vector is vector_name. In the push back method, one element is accepted and the element is inserted at the end of the vector.
Illustration
// CPP program to create an empty vector // and push values one by one. #include <bits/stdc++.h> using namespace std; int main() { // Create an empty vector vector<int> vect; vect.push_back(10); vect.push_back(20); vect.push_back(30); for (int x : vect) cout << x << ” “; return 0; } Output: 10, 20, 30 |
- Using the fill() method
Just as the name implies, it assigns or fills all the elements in the specified range to the specific value. Below is an example of using the fill function.
Illustration
Consider vectors v1 and v2 of size 5.
Input: fill(v1.begin(), v1.end(), 6)
Output: The resulting vector will be:
6 6 6 6 6
Input: fill(v2.begin() + 2, v2.begin() + 4, 6)
Output: The resulting vector will be:
0 0 6 6 0
#include <iostream> #include <vector> using namespace std; int main() { // declare a vector vector<int> v1(5); // initialize the vector using the fill() method fill(v1.begin(), v1.end(), 6); // declare another vector vector<int> v2(5); // initialize v2 fill(v2.begin() + 2, v2.begin() + 4, 6); // print vector elements cout << “The vector elements are: \n”; for (int i = 0; i < v1.size(); i++) { cout << v1[i] << ” “; } cout << “\n\n”; // print vector elements cout << “The vector elements are: \n”; for (int i = 0; i < v2.size(); i++) { cout << v2[i] << ” “; } cout << “\n\n”; return 0; } |
How Do You Initialize Vector Vectors?
In C++ vector initialization, vector vectors are referred to as a two-dimensional vector, and to initialize it, you can first initialize a one-dimensional vector and then use it to initialize a two-dimensional vector or do it in one line. Below is an example of the two.
vector<int> v(5); vector<vector<int> > v2(8,v); |
or
vector<vector<int> > v2(8, vector<int>(5)); |
How to Print a Vector in c++
You can follow these basic codes on how to print a vector in c++
#include<iostream> #include<vector> void print(std::vector <int> const &a) { std::cout << “The vector elements are : “; for(int i=0; i < a.size(); i++) std::cout << a.at(i) << ‘ ‘; } int main() { std::vector<int> a = {2,4,3,5,6}; print(a); return 0; } Output |
Do You Need to Initialize Vectors in C++?
Just like arrays, vectors can store multiple data values. However, they can only store object references and not primitive data types. Storing an object reference means vectors point to the object instead of storing them. Different from arrays, vectors do not need to be initialized with size. They can adjust with respect to the number of object references because storage occurs automatically through the container.
Vectors can be found and transverse with the use of iterators, as such they can be placed in continuous storage. Another property of vector is its safety measure which prevents the program from crashing. A vector is a class and elements can be deleted. But an array is not a class and elements cannot be deleted.
C++ Initialize Vector With Size
In C++, the size of the vector can be initialized when you have a good knowledge of the number of elements that need to be stored. For instance, if you are retrieving data from a database or other sources and you know the number of elements present, let’s say 500, then it is ok to proceed to allocate the vector with an array that can accommodate that amount of data. Although if you don’t know what the size will be, then you can let the vector grow over time.
C++ Initialize Vector to 0
In vector initialization, the number of values in a braced initializer is expected to be less or equal to the number of elements of the vector type. Perhaps there’s any uninitialized element, it will be initialized to zero. Below are examples of vector initialization using the initializer list.
vector unsigned int v1 = {1}; // initialize the first 4 bytes of v1 with 1 // and the remaining 12 bytes with zeros vector unsigned int v2 = {1,2}; // initialize the first 8 bytes of v2 with 1 and 2 // and the remaining 8 bytes with zeros vector unsigned int v3 = {1,2,3,4}; // equivalent to the vector literal // (vector unsigned int) (1,2,3,4) |
Initialize Vector in Constructor
Passing a contractor to vector can be done by following the steps below;
Algorithm Begin Declare a class named as vector. Declare vec of vector type. Declare a constructor of vector class. Pass a vector object v as a parameter to the constructor. Initialize vec = v. Declare a function show() to display the values of vector. for (int i = 0; i < vec.size(); i++) print the all values of variable i. Declare v of vector type. Initialize some values into v in array pattern. Declare ob as an object against the vector class. Pass values of v vector via ob vector object to class vector. Call show() function using vector object to show the all values of vector v. End. |
Initialize 2d Vector C++
Two-dimensional vectors can be grown using several methods. Some of the methods are;
- Use of fill Constructor: This method is the recommended approach. Here, the fill constructor creates a specific number of values. Thereafter, it fills with this value it has created.
- Resize: This method is used to initialize a two-dimensional factor with a given default value.
- Push Back: This method adds a given element to the end of the vector for it to be initialized.
- Use of initializer list: This method involves the use of default values to initialize 2D vectors.
Initialize Empty Vector c++
To initialize empty vector c++, you can follow the steps outlined in the code below.
//C++ STL program to create an empty vector //and initialize by pushing values #include <iostream> #include <vector> using namespace std; int main() { //vector declaration vector<int> v1; //pushing the elements v1.push_back(10); v1.push_back(20); v1.push_back(30); v1.push_back(40); v1.push_back(50); //printing the vector elements //creating iterator to access the elements vector<int>::iterator it; cout << “Vector v1 elements are: “; for (it = v1.begin(); it != v1.end(); it++) cout << *it << ” “; cout << endl; return 0; } |
Conclusion
For effective use of C++, having detailed knowledge of vectors and the process of vector initialization c++ is important. As such, taking your time and indulging in continuous practice should be your goal.
You can maintain consistent practice when you are among a community of like-minded programmers. Join the SDS Club to have access to hundreds of courses and be a part of a growing community of data-driven engineers and scientists.
Subscribe to our newsletter to get real-time updates on posts like this.
Also, share this post with your friends on social media.